
Python Scraper - Google's Cyber Security Daily Top Ten
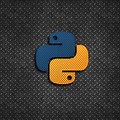
Top News
import requests
def get_top_news():
api_key = "YOUR_API_KEY" # Replace with your News API key
# Specify the API endpoint and parameters
url = "https://newsapi.org/v2/top-headlines"
params = {
"q": "cyber security",
"category": "technology",
"apiKey": api_key,
"pageSize": 10
}
try:
# Send a GET request to the News API
response = requests.get(url, params=params)
data = response.json()
# Check if the request was successful
if response.status_code == 200 and data["status"] == "ok":
articles = data["articles"]
# Display the headlines
for i, article in enumerate(articles, start=1):
title = article["title"]
source = article["source"]["name"]
print(f"{i}. {title} - {source}")
else:
print("Failed to retrieve news articles.")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
# Call the function to get the top news
get_top_news()